Supabase, Server-side rendering and Next.js
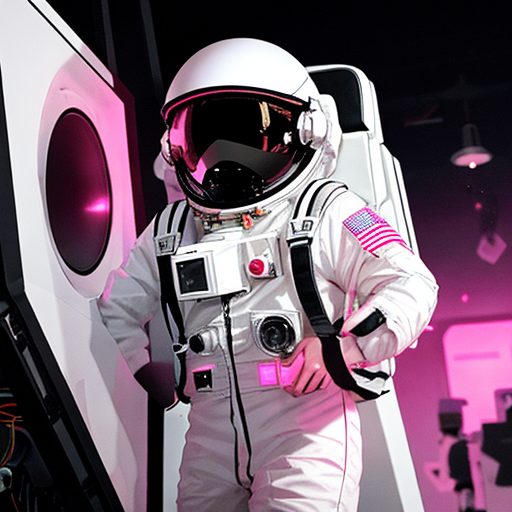
Supabase Server-side Rendering with Next.js 14
In today's digital landscape, delivering fast and interactive user experiences is crucial for the success of any web application. One of the key techniques to achieve this is server-side rendering (SSR), which allows the server to pre-render the HTML content before sending it to the client.
In this blog post, we will explore the powerful combination of Supabase and Next.js 14 to implement server-side rendering in your applications. Supabase is an open-source platform that provides a real-time database and authentication system, making it easier to build scalable and secure applications. Next.js, on the other hand, is a popular React framework that simplifies the process of building server-rendered applications.
We will begin by understanding the basics of Supabase, server-side rendering, and Next.js 14. You will learn how to set up your development environment by installing Node.js and Next.js, as well as configuring Supabase.
Next, we will dive into the integration of Supabase with Next.js 14. You will discover how to connect your Next.js application to Supabase, authenticate users using Supabase's built-in authentication system, and store and retrieve data from the Supabase database.
The main focus of this blog post will be on implementing server-side rendering with Next.js 14. We will discuss the benefits of server-side rendering, and demonstrate how to create server-side rendered pages in Next.js. Additionally, you will learn how to fetch data from Supabase on the server, ensuring that your application's content is pre-rendered and readily available to users.
As we near the end of the blog post, we will explore optimizations to improve the performance of your Next.js application using Next.js optimizations. We will also guide you through the process of deploying your Next.js application with Supabase, and provide tips for monitoring and maintaining your application in the long run.
By the end of this blog post, you will have a solid understanding of how to leverage the power of Supabase, Next.js 14, and server-side rendering to build high-performing and scalable web applications. So, let's get started and unlock the full potential of these cutting-edge technologies!
Understanding the Basics: Supabase, Server-side Rendering, and Next.js 14
Supabase:
Supabase is an open-source platform that provides developers with a set of tools to build scalable and secure applications. It combines the benefits of a real-time database with user authentication and authorization features. With Supabase, you can easily manage your application's data and user authentication in a single solution.
Server-side Rendering (SSR):
Server-side rendering is a technique in web development where the server pre-renders the HTML content of a web page before sending it to the client. This is in contrast to client-side rendering, where the HTML content is generated on the client's browser using JavaScript. SSR offers several advantages, including improved initial page load time, better SEO, and enhanced user experience.
Next.js 14:
Next.js is a popular React framework that simplifies the process of building server-rendered applications. It provides a robust set of features, including server-side rendering, static site generation, and dynamic routing. Next.js 14 is the latest version of the framework, packed with new features and optimizations to enhance the developer experience and improve performance.
In this section, we will delve deeper into the basics of Supabase, server-side rendering, and Next.js 14. Understanding these fundamental concepts is essential before diving into the implementation details of Supabase server-side rendering with Next.js 14. By grasping the core concepts, you will be better equipped to leverage the combined power of Supabase and Next.js 14 to create high-performing and scalable web applications.
Setting Up Your Development Environment
Setting up your development environment is the first step towards building Supabase server-side rendering with Next.js 14. This section will guide you through the necessary steps to ensure your environment is properly configured and ready for development.
Setting Up Supabase
You'll need to set up Supabase for your project. Supabase provides a simple and intuitive interface to manage your application's data and authentication. To get started, visit the Supabase website and sign up for an account. Once you've created an account, you can create a new project and obtain the necessary credentials, including the API URL and your project's public and private keys.
Creating Your Next.js Application
With Node.js, Next.js, and Supabase set up, you're now ready to create your Next.js application. Open your terminal or command prompt and navigate to the directory where you want to create your project. Run the following command to initialize a new Next.js application:
npx create-next-app my-app
Integrating Supabase with Next.js 14
Integrating Supabase with Next.js 14 is a crucial step in building applications that leverage the power of real-time databases and authentication. In this section, we will explore the steps required to connect your Next.js application to Supabase and utilize its features seamlessly.
Connecting Your Next.js App to Supabase
To establish a connection between your Next.js application and Supabase, you will need to install the Supabase client library. Open your terminal or command prompt, navigate to your Next.js project directory, and run the following command:
npm install @supabase/ssr
Next, you need to create a Supabase client instance by providing your project's API URL and public key. You can obtain these credentials from your Supabase project dashboard. Add the following code snippet to initialize the client:
const supabase = createBrowserClient('YOUR_API_URL', 'YOUR_PUBLIC_KEY');
Replace 'YOUR_API_URL'
and 'YOUR_PUBLIC_KEY'
with the actual values from your Supabase project, or change these to process.env.NEXT_PUBLIC_SUPABASE_URL and process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY
Authenticating Users with Supabase
Supabase provides a built-in authentication system that allows you to handle user registration, login, and session management effortlessly. To enable authentication in your Next.js application, you need to configure Supabase and implement the necessary authentication methods.
First, ensure that you have created the required user tables in your Supabase project. You can do this by navigating to the "Authentication" section on your project dashboard and following the prompts to create the necessary tables.
Next, you can implement user registration and login functionality in your Next.js application using the Supabase client methods. For example, you can use the supabase.auth.signUp
method to handle user registration and the supabase.auth.signIn
method to handle user login.
To maintain user sessions, you can use the supabase.auth.session
method to check if a user is authenticated and retrieve their session details.
Storing and Retrieving Data with Supabase
With Supabase integrated into your Next.js application, you can now store and retrieve data from the Supabase real-time database. Supabase provides a client API that allows you to interact with the database using simple and intuitive methods.
To store data, you can use the supabase.from
method to select a table and perform database operations such as inserting, updating, and deleting records. For example:
const { data, error } = await supabase.from('todos').insert({ title: 'My Todo' });
To retrieve data, you can use the supabase.from
method with the select
modifier to specify the columns you want to fetch. You can also apply filters, ordering, and pagination to your queries. For example:
const { data, error } = await supabase.from('todos').select('*').eq('user_id', '123');
By integrating Supabase with Next.js 14, you can take advantage of its real-time database and authentication system to build dynamic and secure applications. In the next section, we will explore how to implement server-side rendering in your Next.js application, further enhancing its performance and user experience.
Implementing Server-side Rendering with Next.js 14
Implementing server-side rendering (SSR) in your Next.js application is a powerful technique that enhances the performance and user experience of your web application. In this section, we will explore the steps required to implement server-side rendering with Next.js 14 and leverage the benefits it offers.
Understanding the Need for Server-side Rendering
Before diving into the implementation details, it's essential to understand why server-side rendering is beneficial for your application. Server-side rendering allows the server to pre-render the HTML content of a web page before sending it to the client. This results in faster initial page load times, improved SEO, and better user experience, especially on slower networks or devices.
By implementing server-side rendering in your Next.js application, you can ensure that the initial HTML content is readily available to users, reducing the time spent waiting for JavaScript to load and render the content on the client-side.
Creating Server-side Rendered Pages in Next.js
Next.js makes it straightforward to create server-side rendered pages in your application. By default, Next.js uses a hybrid rendering approach, where some pages are statically generated and others are server-rendered. You can control the rendering method for each page by using Next.js's page-level data fetching functions.
To create a server-side rendered page, you can export an async
function called getServerSideProps
from your page component. This function runs on the server and fetches the required data for the page. The data returned from this function is then passed as props to your page component.
For example, consider a page that displays a list of blog posts. You can define the getServerSideProps
function as follows:
export async function getServerSideProps() {
// Fetch data from Supabase or any other data source
const res = await fetch('https://api.example.com/posts');
const posts = await res.json();
// Return the data as props
return {
props: {
posts,
},
};
}
The posts
data will now be available as props in your page component, allowing you to render the content on the server and send it as HTML to the client.
Fetching Data from Supabase on the Server
To fetch data from Supabase on the server, you can utilize the Supabase client library within the getServerSideProps
function. This allows you to fetch data from Supabase and include it in the server-side rendered page.
For example, you can modify the previous getServerSideProps
function to fetch blog posts from Supabase:
export async function getServerSideProps() {
const supabase = createClient('YOUR_API_URL', 'YOUR_PUBLIC_KEY');
const { data: posts, error } = await supabase.from('posts').select('*');
return {
props: {
posts,
},
};
}
By leveraging the Supabase client library within the getServerSideProps
function, you can fetch data from Supabase on the server and include it in your server-side rendered page.
Implementing server-side rendering with Next.js 14 and Supabase enables you to deliver faster and more interactive web applications. In the next section, we will explore optimizations to further improve the performance of your Next.js application and guide you through the deployment process.
Optimizing and Deploying Your Application
Optimizing and deploying your Next.js application is a crucial step to ensure optimal performance and make your application accessible to users. In this final section, we will explore various optimizations you can implement and guide you through the deployment process.
Improving Performance with Next.js Optimizations
Next.js provides several optimizations that can significantly improve the performance of your application. Some key optimizations include:
-
Code Splitting: Next.js automatically code-splits your application, which means that only the necessary JavaScript and CSS code is loaded on each page. This improves initial load times and reduces the overall bundle size.
-
Image Optimization: Next.js provides built-in image optimization capabilities. By leveraging the
next/image
component, you can automatically optimize and deliver images in the most efficient format and size for each user's device. -
Caching and Data Fetching Strategies: Next.js offers different data fetching strategies, such as static generation, server-side rendering, and client-side rendering. Choosing the appropriate strategy for each page can optimize performance and ensure the best user experience.
-
Performance Monitoring: Utilize tools like Lighthouse or Next.js Analytics to monitor the performance of your application and identify areas for improvement. These tools can provide insights into metrics such as load times, resource sizes, and accessibility.
By implementing these optimizations, you can enhance the performance of your Next.js application and deliver a seamless user experience.
Deploying Your Next.js Application with Supabase
Once you have optimized your Next.js application, it's time to deploy it to a production environment. Next.js supports various deployment options, including popular hosting platforms like Vercel, Netlify, and AWS Amplify.
To deploy your Next.js application with Supabase, you can follow these general steps:
-
Set up a hosting platform: Choose a hosting platform that supports Next.js deployments, such as Vercel. Sign up for an account and create a new project.
-
Connect your repository: Link your hosting platform to your repository where your Next.js application code resides. This allows for automatic deployments whenever changes are made to your codebase.
-
Configure deployment settings: Configure the deployment settings, including the build command and environment variables required for your application to connect with Supabase.
-
Trigger the deployment: Once the settings are configured, trigger the deployment process. The hosting platform will build your Next.js application, optimize it, and make it available to users.
-
Set up Supabase environment variables: In your hosting platform's dashboard, add the necessary environment variables to connect your deployed Next.js application with Supabase. These variables typically include the Supabase API URL and keys.
-
Test and verify: After the deployment is complete, thoroughly test your application to ensure everything is functioning as expected. Verify that the integration with Supabase is working correctly.
By following these steps, you can successfully deploy your Next.js application with Supabase and make it accessible to users.
Monitoring and Maintaining Your Application
Once your Next.js application is deployed, it's essential to monitor and maintain its performance and functionality. Regularly monitor your application's performance metrics, error logs, and user feedback to identify and address any issues that may arise.
Additionally, it's crucial to keep your dependencies and libraries up to date to benefit from the latest bug fixes and security patches. Regularly review and update your codebase to maintain a secure and efficient application.
Remember to also stay updated with the latest releases and best practices of Next.js and Supabase. Join relevant communities, forums, or mailing lists to stay informed about new features, improvements, and any potential breaking changes.
By monitoring and maintaining your Next.js application, you can ensure its long-term stability, security, and optimal performance.
Congratulations! You have successfully learned how to optimize and deploy your Next.js application with Supabase. By implementing these best practices and continuously improving your application, you can provide users with a high-quality experience while leveraging the power of Supabase and Next.js 14.